此处到处是高手,发个很简单的c#程序,见笑了。
1. 程序说明: 在autocad中打开一个位图,程序将每一个像素用圆绘制出来。
程序作用:无聊游戏之作,不过也是以前一个位图矢量化的初步设想,假如对于黑白线条位图,可用此绘出后,查找圆心,对圆心距离小于sqrt(2)单位的进行连线,以后再进行抽点等操作。当然对于复杂实体和文字等,还需要再寻找算法。
2. 开发平台 visual studio 2005中文版+ autocad 2007英文版。acmgd.dll等的位置是在d:program filesautocad 2007。
3. 程序使用:用Netload导入form1.dll,然后键入命令 my
需要注意的是,由于每个像素绘制一个圆,所以,本程序是没有图片大小限制的,但是假若图片大于250*250像素的时候,需要比较长的时间(我导入的750*350的图片,大概需要2分钟,我是P4 2.8G的CPU),会导致CPU运行满载的情况,请选择图片的时候选择小图片。
4.一些基本代码
复制代码
1. 程序说明: 在autocad中打开一个位图,程序将每一个像素用圆绘制出来。
程序作用:无聊游戏之作,不过也是以前一个位图矢量化的初步设想,假如对于黑白线条位图,可用此绘出后,查找圆心,对圆心距离小于sqrt(2)单位的进行连线,以后再进行抽点等操作。当然对于复杂实体和文字等,还需要再寻找算法。
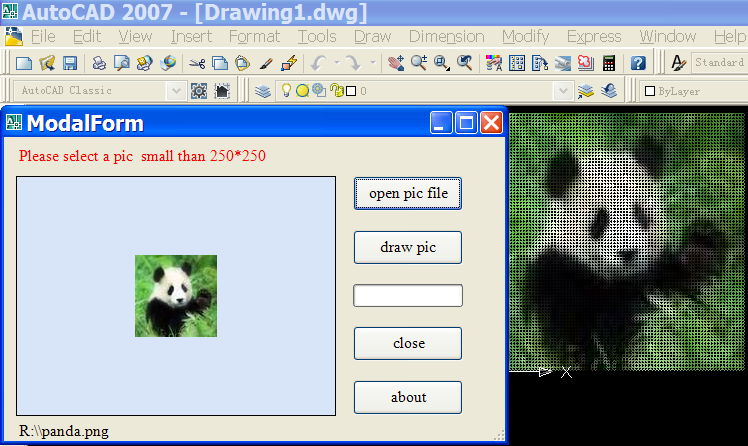
2. 开发平台 visual studio 2005中文版+ autocad 2007英文版。acmgd.dll等的位置是在d:program filesautocad 2007。
3. 程序使用:用Netload导入form1.dll,然后键入命令 my
需要注意的是,由于每个像素绘制一个圆,所以,本程序是没有图片大小限制的,但是假若图片大于250*250像素的时候,需要比较长的时间(我导入的750*350的图片,大概需要2分钟,我是P4 2.8G的CPU),会导致CPU运行满载的情况,请选择图片的时候选择小图片。
4.一些基本代码
- // by qjchen
- // chenqj.blogspot.com
- // 2009-3-27 9:33:23
- using System;
- using System.Collections.Generic;
- using System.ComponentModel;
- using System.Data;
- using System.Drawing;
- using System.Text;
- using System.Windows.Forms;
- using System.IO;
- using form1;
- namespace form1
- {
- using Autodesk.AutoCAD.Runtime;
- using Autodesk.AutoCAD.ApplicationServices;
- using Autodesk.AutoCAD.Colors;
- using Autodesk.AutoCAD.DatabaseServices;
- using Autodesk.AutoCAD.Geometry;
- using Autodesk.AutoCAD.EditorInput;
- using Autodesk.AutoCAD.Internal;
- using Autodesk.AutoCAD.Interop;
- using AcadApp = Autodesk.AutoCAD.ApplicationServices.Application;
- public partial class ModalForm : Form
- {
- public ModalForm()
- {
- InitializeComponent();
- }
- private void button1_Click(object sender, EventArgs e)
- {
- try
- {
- Bitmap myBitmap = new Bitmap(label1.Text);
- System.Drawing.Color c1;
- int intWidth = myBitmap.Width;
- int intHeight = myBitmap.Height;
- if (intWidth * intHeight > 100000)
- {
- MessageBox.Show("It is a big pic" + intHeight + "," + intWidth);
- }
- for (int i = 0; i <= intWidth - 1; i++)
- {
- for (int j = 0; j <= intHeight - 1; j++)
- {
- this.progressBar1.Value = (int)(i) * 100 / intWidth;
- c1 = myBitmap.GetPixel(i, j);
- int cr = c1.R;
- int cg = c1.G;
- int cb = c1.B;
- ObjectId entId = ModelSpace.AddCircle(new Point3d(i, (intHeight - j), 0), 0.5);
- Database db = HostApplicationServices.WorkingDatabase;
- using (Transaction trans = db.TransactionManager.StartTransaction())
- {
- Entity ent = (Entity)trans.GetObject(entId, OpenMode.ForWrite);
- ent.Color = Color.FromRgb((byte)cr, (byte)cg, (byte)cb);
- trans.Commit();
- }
- }
- }
- AcadApplication pApp;
- pApp = (Autodesk.AutoCAD.Interop.AcadApplication)Autodesk.AutoCAD.ApplicationServices.Application.AcadApplication;
- pApp.ZoomExtents();
- }
- catch { }
- }
- private void button2_Click(object sender, EventArgs e)
- {
- OpenFileDialog ofdPic = new OpenFileDialog();
- ofdPic.Filter = "JPG(*.JPG;*.JPEG);gif file(*.GIF);bmp file(*.BMP);png file(*.PNG)|*.jpg;*.jpeg;*.gif;*.bmp;*.png";
- ofdPic.FilterIndex = 1;
- ofdPic.RestoreDirectory = true;
- ofdPic.FileName = "";
- if (ofdPic.ShowDialog() == DialogResult.OK)
- {
- string sPicPaht = ofdPic.FileName.ToString();
- FileInfo fiPicInfo = new FileInfo(sPicPaht);
- long lPicLong = fiPicInfo.Length / 1024;
- string sPicName = fiPicInfo.Name;
- string sPicDirectory = fiPicInfo.Directory.ToString();
- string sPicDirectoryPath = fiPicInfo.DirectoryName;
- Bitmap bmPic = new Bitmap(sPicPaht);
- Point ptLoction = new Point(bmPic.Size);
- if (ptLoction.X > picBox.Size.Width || ptLoction.Y > picBox.Size.Height)
- {
- picBox.SizeMode = PictureBoxSizeMode.Zoom;
- }
- else
- {
- picBox.SizeMode = PictureBoxSizeMode.CenterImage;
- }
- picBox.LoadAsync(sPicPaht);
- label1.Text = sPicDirectoryPath + @"" + sPicName;
- }
- }
- private void button3_Click(object sender, EventArgs e)
- {
- this.Close();
- }
- private void button4_Click(object sender, EventArgs e)
- {
- MessageBox.Show(" To draw bitmap into Autocad by each pixels in circle.n by qjchenn chenqj.blogspot.com");
- }
- [CommandMethod("my")]
- public void drawpic()
- {
- ModalForm modalForm = new ModalForm();
- Application.ShowModalDialog(modalForm);
- }
- }
- }
相关文章
- 2021-09-08BIM技术丛书Revit软件应用系列Autodesk Revit族详解 [
- 2021-09-08全国专业技术人员计算机应用能力考试用书 AutoCAD2004
- 2021-09-08EXCEL在工作中的应用 制表、数据处理及宏应用PDF下载
- 2021-08-30从零开始AutoCAD 2014中文版机械制图基础培训教程 [李
- 2021-08-30从零开始AutoCAD 2014中文版建筑制图基础培训教程 [朱
- 2021-08-30电气CAD实例教程AutoCAD 2010中文版 [左昉 等编著] 20
- 2021-08-30电影风暴2:Maya影像实拍与三维合成攻略PDF下载
- 2021-08-30高等院校艺术设计案例教程中文版AutoCAD 建筑设计案例
- 2021-08-29环境艺术制图AutoCAD [徐幼光 编著] 2013年PDF下载
- 2021-08-29机械AutoCAD 项目教程 第3版 [缪希伟 主编] 2012年PDF